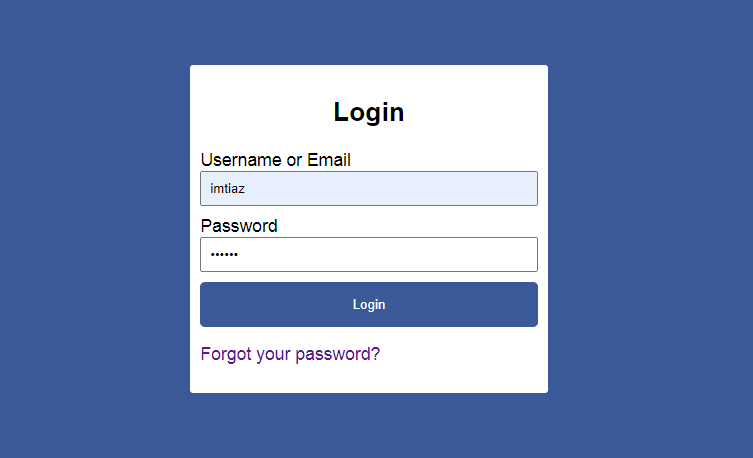
What is a forgotten password and how to recover it?
We are going to learn about how to recover forgot password by sending email to the user using PHP and MySQL. Forgot password recovery is a compulsory feature for the login system. It helps the user to recover the password which they have forgotten by sending a link via email. Using forgot password link user can easily reset their account password. In this tutorial, we create a script to implement forgot password functionality by email in PHP and MySQL.
Before you initiate with this forgot password recovery tutorial, we suggest going through the Our previous tutorials had provided a guide about PHP Login, Logout system using google Recaptcha, and how to add Remember Me functionality in login logout system using PHP and MySQL. So let’s start step by step.
STEPS
We need to follow the following steps below to recover the forgotten password.
Create a directory to recover forgot password.
Example image for the directory.
It is a good practice to create the required folders on your computer so you can proceed and manage it easily.
- CSS folder for custom styling and bootstrap library to make login form beautiful.
- Js folder for jQuery and bootstrap libraries.
Once you have created the required folders it is time to create your HTML structure for login form, send email form and reset password form.
- Launch your text editor and create a new file and save it as "index.php" in your main folder.
- Create a new file and save it as " logic.php" in your main folder.
- Create a new file and save it as "enter_email.php" in your main folder.
- Create a new file and save it as "login.php " in your main folder.
- Create a new file and save it as " status.php " in your main folder.
- Create a new file and save it as "new_pass.php" in your main folder.
- Create a new file and save it as "pending.php" in your main folder.
Create a Database to recover the forgotten password.
Open your phpMyAdmin and create a database with the name (recovery) and create tables in it using the below query.
Table Users.
Table users that store the user’s name, email address and password.
Table users that store the user’s name, email address and password.
--
-- Table structure for table `users`
--
CREATE TABLE IF NOT EXISTS `users` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`email` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`password` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci AUTO_INCREMENT=2 ;
--
-- Dumping data for table `users`
--
INSERT INTO `users` (`id`, `name`, `email`, `password`) VALUES
(1, 'imtiaz', 'test@test.com', '$2y$10$a.ZqYZDmmjSYgm4hm65oYeUnjN7TE2ohNMPUu7l7gbxTNp0Udqn6O');
Table Password_reset.
--
-- Table structure for table `password_reset`
--
CREATE TABLE IF NOT EXISTS `password_reset_request` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`user_id` int(10) unsigned NOT NULL,
`date_requested` datetime NOT NULL,
`token` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci AUTO_INCREMENT=1 ;
In the above table, we add a filed with the name (token) A token that will help us to verify the user making the request. Without this token, the forgot password form would be extremely easy to hack.
You no need to add dummy entry in that tables just you need to use the below username and password for the login form to enter in index.php page.
Username: imtiaz
Password: Imtiaz
Now we are going to create a login form with the forgot password feature in our (login.php) file. If you click on the login button then you will be redirected to the (index.php) page. If you click on forgot password! you will be redirected to the (enter_email) page that we create after creating this login form.
Here is HTML code for login form and forgot Password feature.
<!—logic.php has the control of login,reset pass and send email-->
<?php include('logic.php'); ?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Password Reset PHP</title>
<link rel="stylesheet" href="css/main.css">
</head>
<body>
<form class="login-form" action="login.php" method="post">
<h2 class="form-title">Login</h2>
<!-- form validation status -->
<?php include(status.php'); ?>
<div class="form-group">
<label>Username or Email</label>
<input type="text" value="<?php echo $user_id; ?>" name="user_id">
</div>
<div class="form-group">
<label>Password</label>
<input type="password" name="password">
</div>
<div class="form-group">
<button type="submit" name="login_user" class="login-btn">Login</button>
</div>
<p><a href="enter_email.php">Forgot your password?</a></p>
</form>
</body>
</html>
Your login form looks like as shown below.
Now we create an enter email form in our (enter_email.php) file for the purpose of sending email to the user for resetting the forgotten password.
Here is HTML code for the enter_email form to recover the forgot password.
<!—logic.php has the control of login,reset pass and send email-->
<?php include('logic.php'); ?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Password Reset PHP</title>
<link rel="stylesheet" href="css/main.css">
</head>
<body>
<form class="login-form" action="enter_email.php" method="post">
<h2 class="form-title">Reset password</h2>
<!-- form validation status -->
<?php include(status.php'); ?>
<div class="form-group">
<label>Your email address</label>
<input type="email" name="email">
</div>
<div class="form-group">
<button type="submit" name="reset-password" class="login-btn">Submit</button>
</div>
</form>
</body>
</html>
Enter_email form looks like as shown below.
After entering the email address in this form and clicking the submit button you received a message of sending an email to the user. Open the email account and click on the link that you have received in email and reset your password.
Now we create a reset password form in our (new_pass.php) file for the purpose of creating a new password.
Here is HTML code for reset password form.
<?PHP include('logic.php');?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Password Reset PHP</title>
<link rel="stylesheet" href="css/main.css">
</head>
<body>
<form class="login-form" action="new_pass.php" method="post">
<h2 class="form-title">New password</h2>
<!-- form validation status -->
<?php include(status.php'); ?>
<div class="form-group">
<label>New password</label>
<input type="password" name="new_pass">
</div>
<div class="form-group">
<label>Confirm new password</label>
<input type="password" name="new_pass_c">
</div>
<div class="form-group">
<button type="submit" name="new_password" class="login-btn">Submit</button>
</div>
</form>
</body>
</html>
The reset password form looks like as shown below.
Now we create a status messages script in our (status.php) file by adding the below code in it for showing the messages of errors and successful.
Here is the code for showing the status messages.
<?php if (count($errors) > 0) : ?>
<div class="msg">
<?php foreach ($errors as $error) : ?>
<span><?php echo $error ?></span>
<?php endforeach ?>
</div>
<?php endif ?>
Now we create a pending form in our (pending.php) file by adding the below code in it. Basically this file contains on the message that we received after entering the email in (enter_email.php) form.
<?PHP include('logic.php');?>
<html>
<head>
<meta charset="UTF-8">
<title>Password Reset PHP</title>
<link rel="stylesheet" href="css/main.css">
</head>
<body>
<form class="login-form" action="login.php" method="post" style="text-align: center;">
<p>
We sent an email to <b><?php echo $_GET['email'] ?></b> to help you recover your account.
</p>
<p>Please login into your email account and click on the link we sent to reset your password</p>
</form>
</body>
</html>
Your status message looks like as shown below.
Now we create an index page in our (index.php) file and write the below code in it. After successful login you will be redirected to this page.
<html>
<head>
<title>Welcome</title>
<link href="css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css">
</head>
<style>
body {
background-image: url("css/images/bg-image.jpg");
background-repeat: no-repeat;
background-attachment: fixed;
width: 100%;
height: 100%;
background-size: cover;
background-color: #cccccc;
}
h1 { background-color: aqua;
}
</style>
<body>
<h1>Welcome to uvisionpk.com Software and Web Development Company.</h1>
<!--bootstrap library-->
<script src="js/bootstrap.min.js"></script>
<!--jquery library-->
<script src="js/jquery.min.js"></script>
</body>
</html>
Finally, we are going to write a PHP script for recovering the forgotten password by sending an email in our (logic.php) file this file has the control of the login form, reset password form, enter email form mean all above HTML forms that we have created. This is a simple and cool script you can easily understand it.
Here is the PHP script to recover forgot password.
<?php
session_start();
$errors = [];
$user_id = "";
// connect to database
$db = mysqli_connect('localhost:', 'root', '', 'password_recovery');
// LOG USER IN
if (isset($_POST['login_user'])) {
// Get username and password from login form
$user_id = mysqli_real_escape_string($db, $_POST['user_id']);
$password = mysqli_real_escape_string($db, $_POST['password']);
// validate form
if (empty($user_id)) array_push($errors, "Username or Email is required");
if (empty($password)) array_push($errors, "Password is required");
// if no error in form, log user in
if (count($errors) == 0) {
$password = md5($password);
$sql = "SELECT * FROM users WHERE username='$user_id' OR email='$user_id' AND password='$password'";
$results = mysqli_query($db, $sql);
if (mysqli_num_rows($results) == 1) {
$_SESSION['username'] = $user_id;
$_SESSION['success'] = "You are now logged in";
header('location: index.php');
}else {
array_push($errors, "Wrong credentials");
}
}
}
/*
Accept email of user whose password is to be reset
Send email to user to reset their password
*/
if (isset($_POST['reset-password'])) {
$email = mysqli_real_escape_string($db, $_POST['email']);
// ensure that the user exists on our system
$query = "SELECT email FROM users WHERE email='$email'";
$results = mysqli_query($db, $query);
if (empty($email)) {
array_push($errors, "Your email is required");
}else if(mysqli_num_rows($results) <= 0) {
array_push($errors, "Sorry, no user exists on our system with that email");
}
// generate a unique random token of length 100
$token = bin2hex(random_bytes(50));
if (count($errors) == 0) {
// store token in the password-reset database table against the user's email
$sql = "INSERT INTO password_reset(email, token) VALUES ('$email', '$token')";
$results = mysqli_query($db, $sql);
// Send email to user with the token in a link they can click on
$to = $email;
$subject = "Reset your password on examplesite.com";
$msg = "Hi there, click on this <a href=\"new_password.php?token=" . $token . "\">link</a> to reset your password on our site";
$msg = wordwrap($msg,70);
$headers = "From: test@test.com";
mail($to, $subject, $msg, $headers);
header('location: pending.php?email=' . $email);
}
}
// ENTER A NEW PASSWORD
if (isset($_POST['new_password'])) {
$new_pass = mysqli_real_escape_string($db, $_POST['new_pass']);
$new_pass_c = mysqli_real_escape_string($db, $_POST['new_pass_c']);
// Grab to token that came from the email link
$token = $_SESSION['token'];
if (empty($new_pass) || empty($new_pass_c)) array_push($errors, "Password is required");
if ($new_pass !== $new_pass_c) array_push($errors, "Password do not match");
if (count($errors) == 0) {
// select email address of user from the password_reset table
$sql = "SELECT email FROM password_reset WHERE token='$token' LIMIT 1";
$results = mysqli_query($db, $sql);
$email = mysqli_fetch_assoc($results)['email'];
if ($email) {
$new_pass = md5($new_pass);
$sql = "UPDATE users SET password='$new_pass' WHERE email='$email'";
$results = mysqli_query($db, $sql);
header('location: index.php');
}
}
}
?>
To understand the above PHP script read the comments carefully that we have writ in this script.
Remember:
Don’t forget to change the email content, from the header, as per your project requirement.
Now we are going to write some custom CSS for styling of our HTML forms that we have already created, in our (style.css) file that we have already created in our CSS folder.
Here is custom CSS code.
body {
background: #3b5998;
font-size: 1.1em;
font-family: sans-serif;
}
a {
text-decoration: none;
}
form {
width: 25%;
margin: 70px auto;
background: white;
padding: 10px;
border-radius: 3px;
}
h2.form-title {
text-align: center;
}
input {
display: block;
box-sizing: border-box;
width: 100%;
padding: 8px;
}
form .form-group {
margin: 10px auto;
}
form button {
width: 100%;
border: none;
color: white;
background: #3b5998;
padding: 15px;
border-radius: 5px;
}
.msg {
margin: 5px auto;
border-radius: 5px;
border: 1px solid red;
background: pink;
text-align: left;
color: brown;
padding: 10px;
}
Other Related Posts
PHP Login, Logout system using google recaptcha
PHP Remember me feature in login logout using PHP
We have all done. Hopefully, you found this tutorial to be useful! Please share your thoughts and queries in the contact section.
Below is the Download link of the above tutorial.
Click here to download full source code