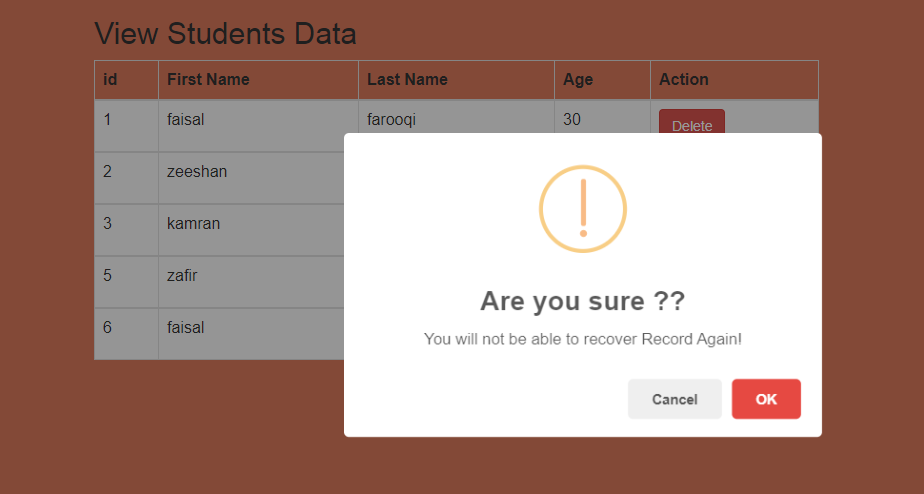
Today we are going to learn about Ajax and how to delete database records using Ajax and jQuery by adding sweet alert in PHP. The main purpose of Ajax is to improve the speed, performance, and usability of a web application. So in this article, we will learn Ajax with an example of deleting the database records using Ajax without refreshing and reloading the web page.
What is an Ajax and why we use it?
Ajax Definition.
Ajax is the method of exchanging information with a server, and updating parts of a web page without refreshing and reloading the whole page.
AJAX stands for Asynchronous JavaScript and XML. XML is another alternative of a markup language like HTML, Ajax is not a programming language or a tool, but a concept. Ajax is a client-side script that works asynchronously – processing any requests to the server in the background. So let’s start step by step.
In this example, I am creating the HTML table which shows the list of records with a delete button. When the button clicked then remove the record and also remove HTML table row.
STEPS
We need to follow the following steps below.
Create directory.
Example image for the directory.
It is a good practice to create the required folders on your computer so you can proceed and manage it easily.
- CSS folder for custom styling of HTML and for storing the bootstrap and sweet alert libraries.
- Includes has the database connection file with name conn.php.
- Js folder for storing the bootstrap and sweet alert libraries.
- Launch your text editor and create a new file and save it as "student.php" in your main folder.
- Create a new file and save it as "ajax_delete.php" in the same place where you store students.php file.
- Create one more new file and save it as "style.css" in the CSS folder.
Create a Database and a Table in it.
Open your phpMyAdmin and create a database with the name (school) and create a table (students) in it using the below query.
CREATE TABLE `students` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`first_name` varchar(255) NOT NULL,
`last_name` varchar(255) NOT NULL,
`age` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1
We have created a simple table in the database with the columns like first_name, last_name, and age.
Create a Database connection.
Create a new file with the name of (conn.php) and Write the below code in it and save it in includes folder.
<?php
$con=mysqli_connect("localhost","root","","school");
?>
Create HTML table and fetch data from Database.
Now open your student.php file and make HTML table to display the student’s records and action button by adding the below html code.
<?php
//For database connection
include "includes/conn.php";
?>
<html>
<head>
<title> </title>
<!--bootstrap libraray-->
<link rel="stylesheet" href="css/bootstrap.min.css">
<!--sweetalert libraray-->
<link href="css/sweetalert.css" rel="stylesheet">
<!--custom css-->
<link rel="stylesheet" type="text/css" href="css/style.css">
</head>
<body>
<div class="content-wrap">
<div class="main">
<div class="container-fluid">
<!-- /# row -->
<div id="main-content" >
<div class="row">
<div class="col-lg-12">
<div class="card alert">
<div class="card-header">
<h2> View Students Data </h2>
<div class="card-header-right-icon">
</div>
</div>
<div class="bootstrap-data-table-panel">
<div class="table-responsive">
<!-- html table -->
<table class="table table-striped table-bordered">
<thead>
<tr>
<th>id</th>
<th>First Name</th>
<th>Last Name</th>
<th>Age</th>
<th>Action</th>
</tr>
</thead>
<?php
//query for retriving the records from database
$query= mysqli_query($con,"select * from students");
foreach($query as $val){
?>
<tbody>
<tr>
<td><?php echo $val["id"];?></td>
<td><?php echo $val["first_name"];?></td>
<td><?php echo $val["last_name"];?></td>
<td><?php echo $val["age"];?></td>
<!--actions buttons for edit and delete-->
<td><a href="#" class="btn btn-danger delete" ><i class="fa fa-trash"></i>Delete</a></td>
</tr>
</tbody>
<?php
}
?>
</table>
</div>
</div>
</div>
<!-- /# card -->
</div>
<!-- /# column -->
</div>
<!-- /# row -->
</div>
</div>
</div>
</div>
<!--bootstrap libraray-->
<script src="js/jquery.min.js"></script>
<script src="js/script.js"></script>
<script src="js/bootstrap.min.js"></script>
<script type="text/javascript" src="js/sweetalert.js"></script>
<body>
</html>
After creating HTML table and fetching the database data in it your table looks as shown below.
You can download the above bootstrap, jQuery, and sweet alert libraries that we use to make HTML table beautiful by clicking the below links. By clicking on the links you will be redirected to the new page. So copy all code and save them in their folders by creating the files and give the name to the file.
https://maxcdn.bootstrapcdn.com/bootstrap/4.1.1/css/bootstrap.min.css
https://maxcdn.bootstrapcdn.com/bootstrap/4.1.1/js/bootstrap.min.js
https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js
https://cdnjs.cloudflare.com/ajax/libs/bootstrap-sweetalert/1.0.1/sweetalert.js
https://cdnjs.cloudflare.com/ajax/libs/bootstrap-sweetalert/1.0.1/sweetalert.min.css
Now we are going to write a jQuery Ajax script to delete the database records without refreshing and reloading the page by getting record id and sending this id by URL to (ajax_delete.php) through the Ajax script.
$(document).ready(function(){
// delete onclick event
$(document).on("click",".delete",function(e){
var id=$(this).parent().parent().children().first().html();
//alert(id);
var thiss=$(this);
e.preventDefault();
swal({
title: "Are you sure ??",
text: "You will not be able to recover Record Again!",
icon: "warning",
buttons: true,
dangerMode: true,
})
.then((willDelete) => {
if (willDelete) {
$.ajax({
url:"ajax_delete.php",
data:{
id:id,
action:"delete_student"
},
type:"post",
dataType:"json",
success:function(response){
thiss.parent().parent().remove();
}
});
swal("Deleted!", "your imaginary file has been deleted.", "success");
}
else{
swal("Cancelled","your imaginary file is safe :)" , "error");
}
});
});
});
JQuery Ajax Script Explanation.
$(document).ready(function(){
This is the JQuery function which runs as soon as the document becomes ready. This is similar to the onload event.
$(document).on("click",".delete",function(e){
Define Click event on delete class when the user clicks on the button that has the deleted class the code will be executed.
var id=$(this).parent().parent().children().first().html();
//alert(id);
var thiss=$(this);
The id variable refers to the parent of this hyperlink which is TD and then this TD’s parent which is TR. So here we get the id of this TR. In HTML code, we will allow each row’s primary key field to these TRs to identify and delete records.
swal({
title: "Are you sure ??",
text: "You will not be able to recover Record Again!",
icon: "warning",
buttons: true,
dangerMode: true,
})
.then((willDelete) => {
if (willDelete) {
We want to be able to confirm if the user really wants to delete a row. The above code show confirmation sweet alert instead of simple jQuery alert.
$.ajax({
url:"ajax_delete.php",
data:{
id:id,
action:"delete_student"
},
type:"post",
dataType:"json",
success:function(response){
thiss.parent().parent().remove();
}
});
swal("Deleted!", "your imaginary file has been deleted.", "success");
}
else{
swal("Cancelled","your imaginary file is safe :)" , "error");
}
});
});
});
- The $.Ajax function is used to send Ajax requests.
- The data variable makes data to be sent as part of the Ajax request made using JQuery.
- The type refers to the method of request whether it POST or GET,
- URL refers to a script which will get the Ajax request data and return some response,
- Success function which also attributes of the $.Ajax function runs the code inside it if the request went successful.
- As explained before parent refers to the TRs in our case so the TRs with the id that we send using URL that will be deleted.
PHP script for deleting the database record by sending a request using Ajax.
Now open the Ajax_delete.php file and type the below PHP script in it.
<?php
//database connection
include "includes/conn.php";
//empty message veriable
$msg="";
$res=array();
if(isset($_session["id"])){
}
//pass the action
if(isset($_POST['action'])&&$_POST['action']=="delete_student"){
$id=mysqli_real_escape_string($con,$_POST['id']);
//delete query
$delet=mysqli_query($con,"delete from `students` WHERE id='$id'")or die(mysqli_error($con)) ;
if(mysqli_affected_rows($con)>0){
//status messages
$msg="<div class='alert alert-success'>student record deleted Successfully</div>";
}
else{
$msg="<div class='alert alert-danger'>Unsuccessfully</div>";
}
$res=array(
"msg"=>$msg
);
//send response to back.
echo json_encode($res);
}
?>
Now open the style.css file from the CSS folder and write the below code in it for styling the body section of HTML table.
.container-fluid {
padding-right: 431px;
padding-left: 178px;
margin-right: auto;
margin-left: auto;
}
body {
font-family: "Helvetica Neue",Helvetica,Arial,sans-serif;
font-size: 14px;
line-height: 1.42857143;
color: #333;
background-color: #da795c;
}
.btn-success {
color: #fff;
background-color: #5cb85c;
border-color: #4cae4c;
margin-left: 600px;
}
.alert h4 {
margin-top: 0;
color: inherit;
text-align: center;
}
Other Related Posts
How to create beautiful alerts using bootstrap sweetalert plugin.
How to add edit and delete records from the database in PHP
We have all done. Now you can delete records from the database using jQuery Ajax without refreshing and reloading the web page. Here is the final output.
Final Output Image.
We hope you may learn how to delete records from the database using Ajax. Please share your thoughts and queries in the contact section.
Below is the Download link of the above tutorial.