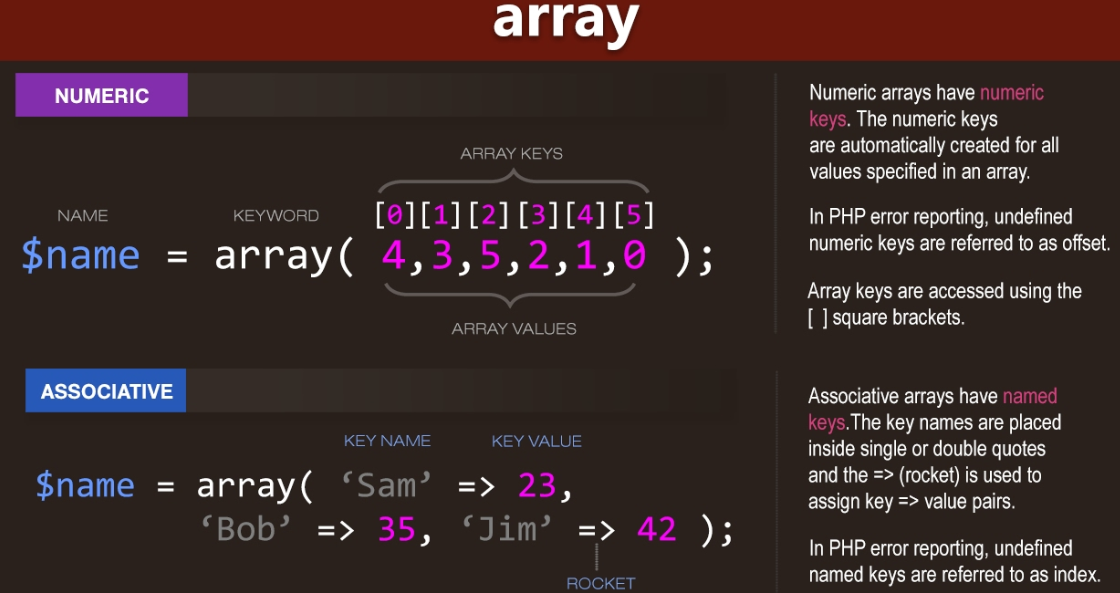
What is PHP Arrays?
In this tutorial, we are going to learn about PHP arrays and their type’s numeric, associative, and multidimensional arrays. PHP arrays are used to store a collection of values in one place instead of creating different variables. Let's assume you want to store different colors in your PHP script. Storing the colors one by one in a variable looks like as shown below.
<?php
$color1 = "Red";
$color2 = "Green";
$color3 = "Blue";
?>
If you want to store the numbers in variables and this time this not just three maybe a hundred or thousand. It is fairly tough, uninteresting, and a bad hint to store each number in a separate variable. Now here are the arrays to avoid creating separate variables.
Types of PHP Array.
There are 3 types of an array in PHP.
- Indexed or Numeric Array
- Associative Array
- Multidimensional Array
PHP numeric or indexed Array.
PHP index is represented by a number which always starts from 0. We can store number, string, and object in the PHP array. All PHP array elements are assigned to an index number by default.
There are two ways to describe the indexed or numeric array.
The first way to create a PHP numeric array.
<?php
/* 1st way to create Numeric array. */
$numeric = array( 1, 2, 3, 4, 5);
foreach( $numeric as $value ) {
echo "Value is $value <br />";
}
?>
Output Of the above PHP numeric arrays.
Value is 2
Value is 3
Value is 4
Value is 5
The second way to create a PHP numeric array.
<?php
/* 2nd way to create Numeric array. */
$numeric[0] = "one";
$numeric[1] = "two";
$numeric[2] = "three";
$numeric[3] = "four";
$numeric[4] = "five";
foreach( $numeric as $value ) {
echo "Value is $value <br />";
}
?>
OutPut Of the above PHP numeric arrays.
Value is two
Value is three
Value is four
Value is five
PHP Associative Array.
PHP Associative Arrays contains the Alphanumeric Index instead of the Numeric Index. Associative array will have their index as string so that you can create a strong relationship between key and values.
To store the salaries of employees in an array. We could use the employee's names as the keys in our associative array, and the value would be their respective salary. A numerically indexed array would not be the best choice.
There are two ways to describe a PHP associative array.
The first way to create a PHP associative array.
<?php
/* 1st method to create associative array. */
$salaries = array("faisal" => 5000, "zafir" => 10000, "kamran" => 15000);
echo "Salary of faisal is ". $salaries['faisal'] . "<br />";
echo "Salary of zafir is ". $salaries['zafir']. "<br />";
echo "Salary of kamran is ". $salaries['kamran']. "<br />";
?>
OutPut Of the above PHP associative arrays.
Salary of zafir is 10000
Salary of kamran is 15000
The second way to create a PHP associative array.
<?php
/* 2nd way to create associative array. */
$salaries['faisal'] = "low";
$salaries['zafir'] = "medium";
$salaries['kamran'] = "high";
echo "Salary of faisal is ". $salaries['faisal'] . "<br />";
echo "Salary of zafir is ". $salaries['zafir']. "<br />";
echo "Salary of kamran is ". $salaries['kamran']. "<br />";
?>
OutPut Of the above PHP associative arrays.
Salary of zafir is medium
Salary of kamran is high
PHP multidimensional array.
PHP multidimensional array is an array in which each element can also be an array and each element in the sub-array can be an array. The advantage of multidimensional arrays is that they allow us to group related data together.
Below is the example of a PHP multidimensional array.
<?php
/* Multidimentional Array */
$obtainmarks = array(
"faisal" => array (
"physics" => 35,
"maths" => 30,
"chemistry" => 39
),
"zafir" => array (
"physics" => 30,
"maths" => 32,
"chemistry" => 29
),
"kamran" => array (
"physics" => 31,
"maths" => 22,
"chemistry" => 39
)
);
/* Accessing multi-dimensional array values */
echo "obtainmarks of faisal in physics : " ;
echo $obtainmarks['faisal']['physics'] . "<br />";
echo "obtainmarks of zafir in maths : ";
echo $obtainmarks['zafir']['maths'] . "<br />";
echo "obtainmarks of kamran in chemistry : " ;
echo $obtainmarks['kamran']['chemistry'] . "<br />";
?>
OutPut Of the above PHP multidimensional arrays.
Obtainmarks of zafir in maths : 32
Obtainmarks of kamran in chemistry : 39
Other Related Posts
How to use PHP for Loop and foreach Loop
How to use PHP while Loop and do while Loop
We hope you may learn how to use PHP numeric array, associative array, and multidimensional array. Please share your thoughts and queries in the contact section.