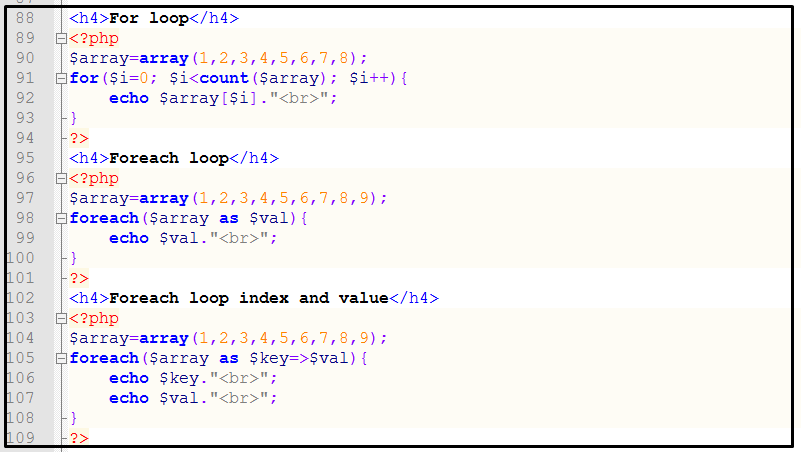
What is PHP Loops and How to use them.
PHP Loops are used to implement the same block of code again and again multiple times until and unless a specific condition is happened. The basic idea behind a PHP loops is to help the users to save both time and effort of writing the same code multiple times.
PHP supports different kinds of loops.
But In this tutorial we will learn about for and foreach loops which are also used to implement looping in PHP programing.
PHP for Loop.
For loop is a control Statement. For loop statement is used when you know how many times you want to execute a code or a block of code.
Syntax of PHP for Loop.
for (init counter; test counter; increment counter) {
code to be executed;
}
The parameters used in for loop syntax have following meaning:
Init counter: it is used to initialize the counter variables, and evaluated once unconditionally before the first execution of the body of the loop.
Test counter: In this parameters we have to test the condition. If the condition is true then we will execute the body of loop and go to update counter otherwise we will exit from the for loop.
Increment counter: Here we increment the loop counter as per the requirements.
Below is the flow chart to understand that how PHP for loop Works?
Hope you understand PHP for loop syntax and its Parameters now we understand this PHP for loop with example. Let’s try to print numbers from 1 to 10.
<?php
for($a = 1; $a <= 10; $a++)
{
echo "$a <br/>";
}
?>
2
3
4
5
6
7
8
9
10
Nested for Loops
We can also use for loop inside another for loop. Below is a simple example of nested for loops.
<?php
for($a = 0; $a <= 2; $a++)
{
for($b = 0; $b <= 2; $b++)
{
echo "$b $a <br/>";
}
}
?>
1 0
2 0
0 1
1 1
2 1
0 2
1 2
2 2
Now we are going to learn about PHP foreach Loop.
PHP foreach Loop.
Foreach loop is a special type of control statement which is used for array. It access all the elements in an array.
Syntax of PHP foreach Loop.
<?php
foreach($array as $value){
/*
Execute this code for all the
Array elements
$value will represent all the array
Elements starting from first element,
One by one
*/
}
?>
Foreach loop pass the value of the current array element is assigned to $value and the array pointer is moved one by one and in the next pass next element will be processed.
Let’s try with simple example.
<?php
$array = array("faisal", "zafir", "kamran", "zeeshan");
foreach($array as $value)
{
echo "$value <br/>";
}
?>
zafir
kamran
zeeshan
Here is one more syntax of PHP foreach loop, which is addition or extension of the first.
Syntax of PHP foreach Loop with extention.
<?php
foreach($array as $key => $value){
// Code to be executed
}
?>
Let’s try with simple example using the above syntax.
<?php
$array = array(
"name" => "Faisal",
"email" => "faisal@gmail.com",
"age" => 26
);
// Loop through array
foreach($array as $key => $value){
echo $key . " : " . $value . "<br>";
}
?>
email : faisal@gmail.com
age : 26
Other Related Posts
How to use PHP while Loop and do while Loop
We hope you may learn how to use PHP for loop and foreach loop. Please share your thoughts and queries in the contact section.